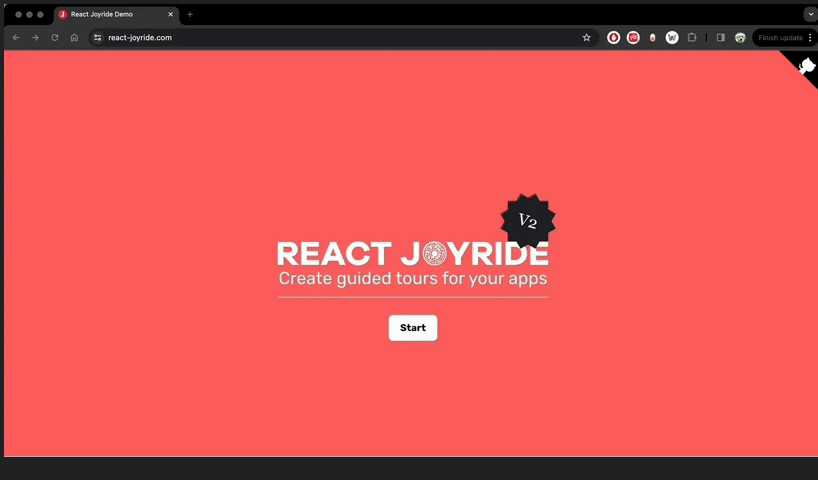
Recently, I researched the commonly used open source libraries for React beginners and found that react-joyride is the most customizable and suitable for in-depth use.
react-joyride official Demo effect:
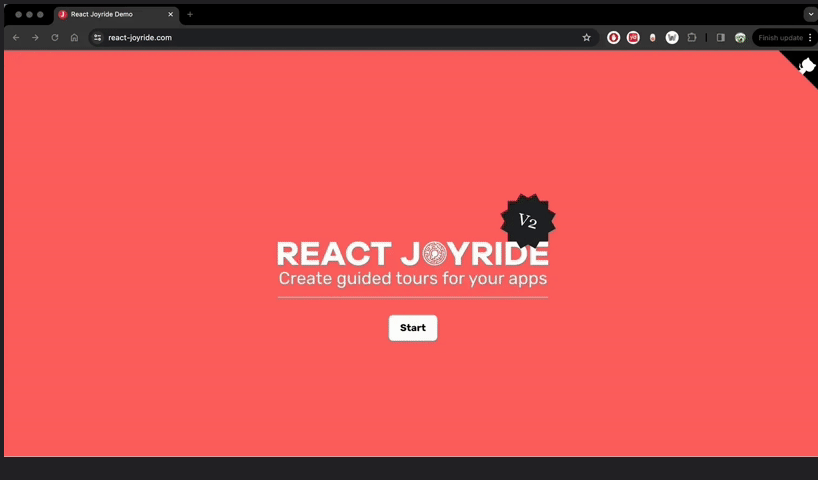
Demo github: https://github.com/gilbarbara/react-joyride
The most downloaded open source libraries for product tour in the past year
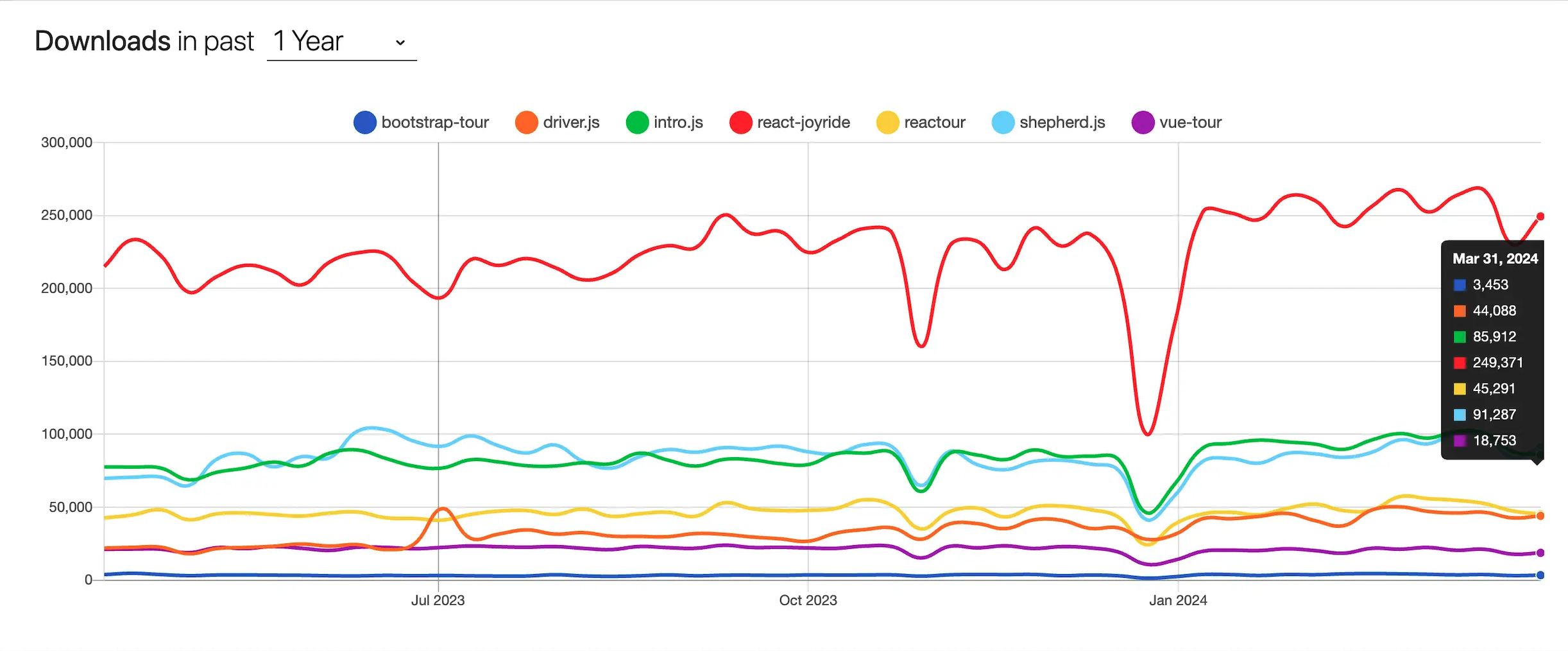
The statistical date is March 31, 2024
Library | Star | NPM daily download | Agreement |
---|---|---|---|
react-joyride | 6.4k | 249k | MIT |
shepherd.js | 11.8k | 91k | MIT |
driver.js | 20.4k | 44k | MIT |
intro.js | 22.6k | 85k | GPL |
reactour | 3.7k | 45k | MIT |
- From the daily download volume of npm, react-joyride is far ahead, with more than 2.5 times the download volume of the second place shepherd.js.
- intro.js is a GPL agreement. If you do not purchase its commercial agreement and use it in enterprise applications, you must open the relevant source code and be very careful when using it 📢
Quick Start 🚀
Step 1: Import
import React from 'react';
import Joyride from 'react-joyride';
export default function App() {
return <Joyride steps={steps} />;
}
Step 2: Define the boot steps
const steps = [
{
target: '.my-first-step',
content: 'This is my awesome feature!',
},
{
target: '.my-other-step',
content: 'This another awesome feature!',
},
];
<Joyride steps={steps} />;
React and TypeScript support 🏡
-
react-joyride source code is implemented using React and TypeScript, with the highest support
-
The intro.js source code is implemented using TypeScript, not React.
-
The shepherd.js source code is implemented using React and JavaScript, does not support TypeScript, and has no export declaration file
-
reactour supports React Hooks, the source code is implemented in JavaScript, and supports TypeScript type declarations
-
driver.js source code is implemented using TypeScript, and does not use React
Custom styles 🌺
Custom styles are standard in the above novice guide library and are all supported. Take react-joyride as an example
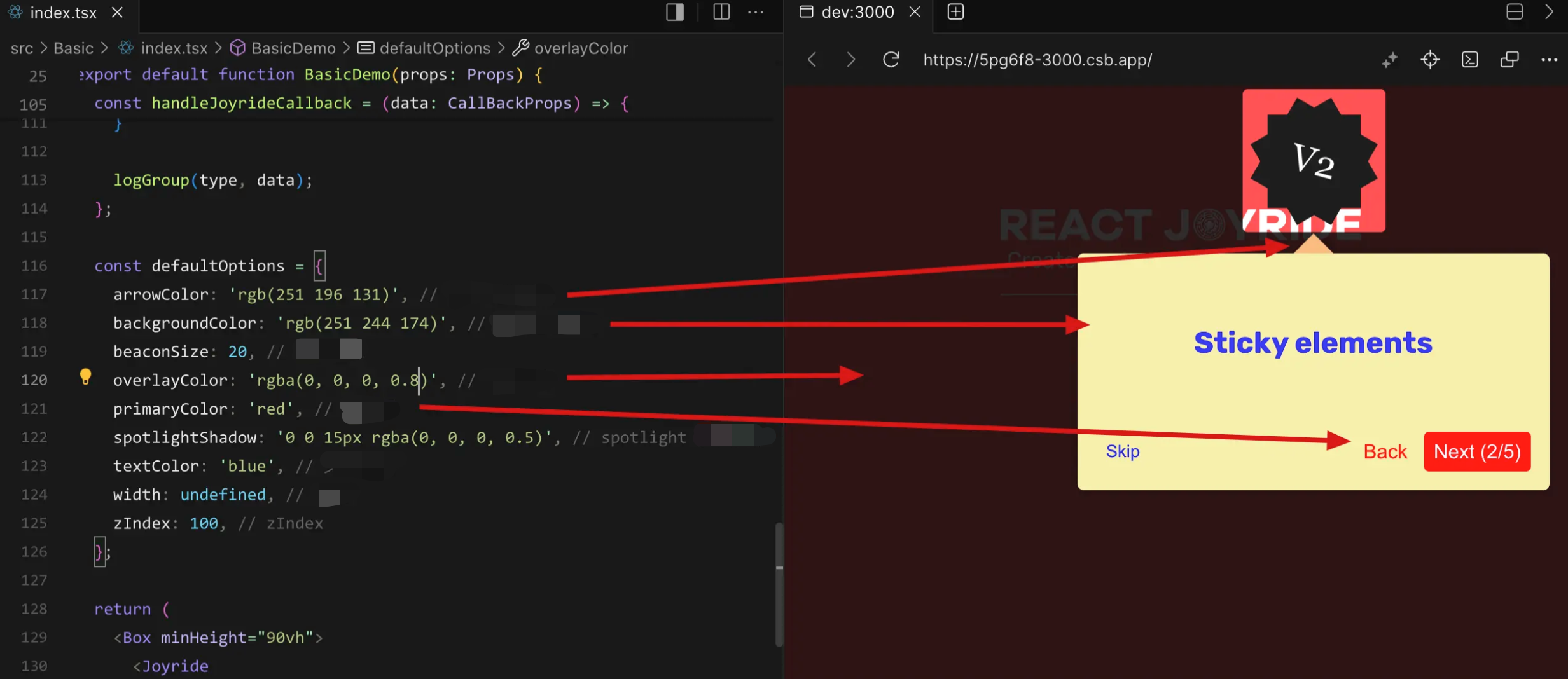
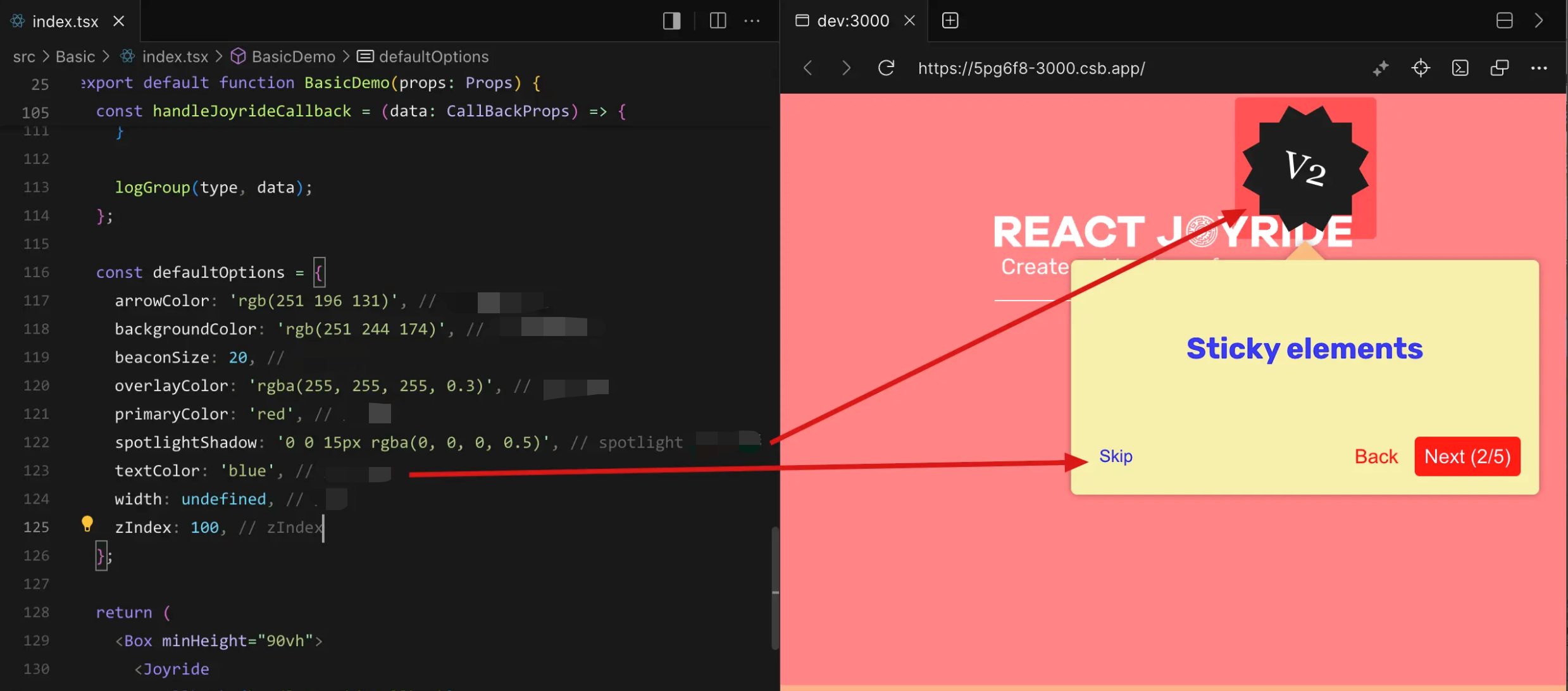
const customOptions = {
arrowColor: 'rgb(251 196 131)',
backgroundColor: 'rgb(251 244 174)',
beaconSize: 20,
overlayColor: 'rgba(0, 0, 0, 0.8)',
primaryColor: 'red',
spotlightShadow: '0 0 15px rgba(0, 0, 0, 0.5)',
textColor: 'blue',
width: undefined,
zIndex: 100, // zIndex
};
<Joyride
callback={handleJoyrideCallback}
continuous
hideCloseButton
run={run}
scrollToFirstStep
showProgress
showSkipButton
steps={steps}
styles={{
options: customOptions,
}}
/>;
Custom Bootstrap Components 🔧
Custom React components are a unique feature of react-joyride, which other libraries do not have. shepherd.js and driver.js support JavaScript to operate HTML elements and overwrite CSS Class styles, which are difficult to develop and maintain (from the MVVM era back to the HTML+JS+CSS era →→)
Custom component
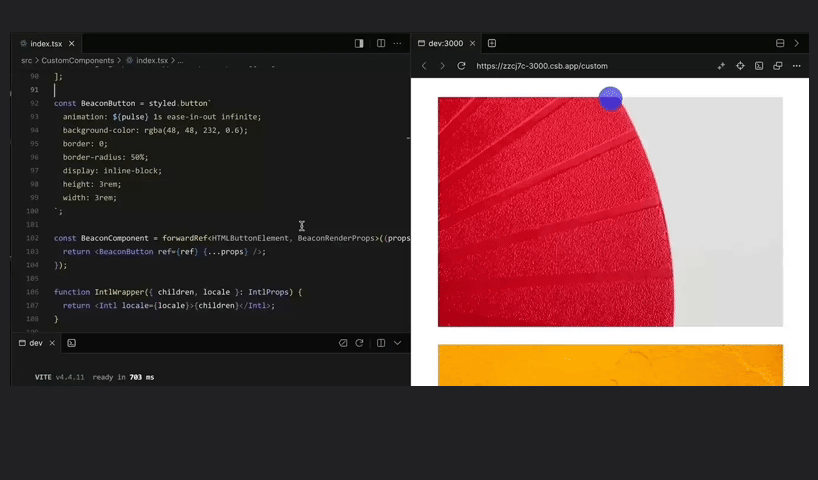
import Joyride, { BeaconRenderProps, TooltipRenderProps } from 'react-joyride';
const pulse = keyframes`
0% {
transform: scale(1);
}
55% {
background-color: rgba(255, 100, 100, 0.9);
transform: scale(1.6);
}
`;
const Beacon = styled.span`
animation: ${pulse} 1s ease-in-out infinite;
background-color: rgba(255, 27, 14, 0.6);
border-radius: 50%;
display: inline-block;
height: 3rem;
width: 3rem;
`;
const BeaconComponent = forwardRef<HTMLButtonElement, BeaconRenderProps>((props, ref) => {
return <BeaconButton ref={ref} {...props} />;
});
export () => (
<div>
<ReactJoyride
beaconComponent={BeaconComponent}
// beaconComponent={BeaconComponent as React.ElementType<BeaconRenderProps>} for TS
...
/>
</div>
);
Detailed code address: https://react-joyride.com/custom
Customizing the Tooltip Component
Default Tooltip component
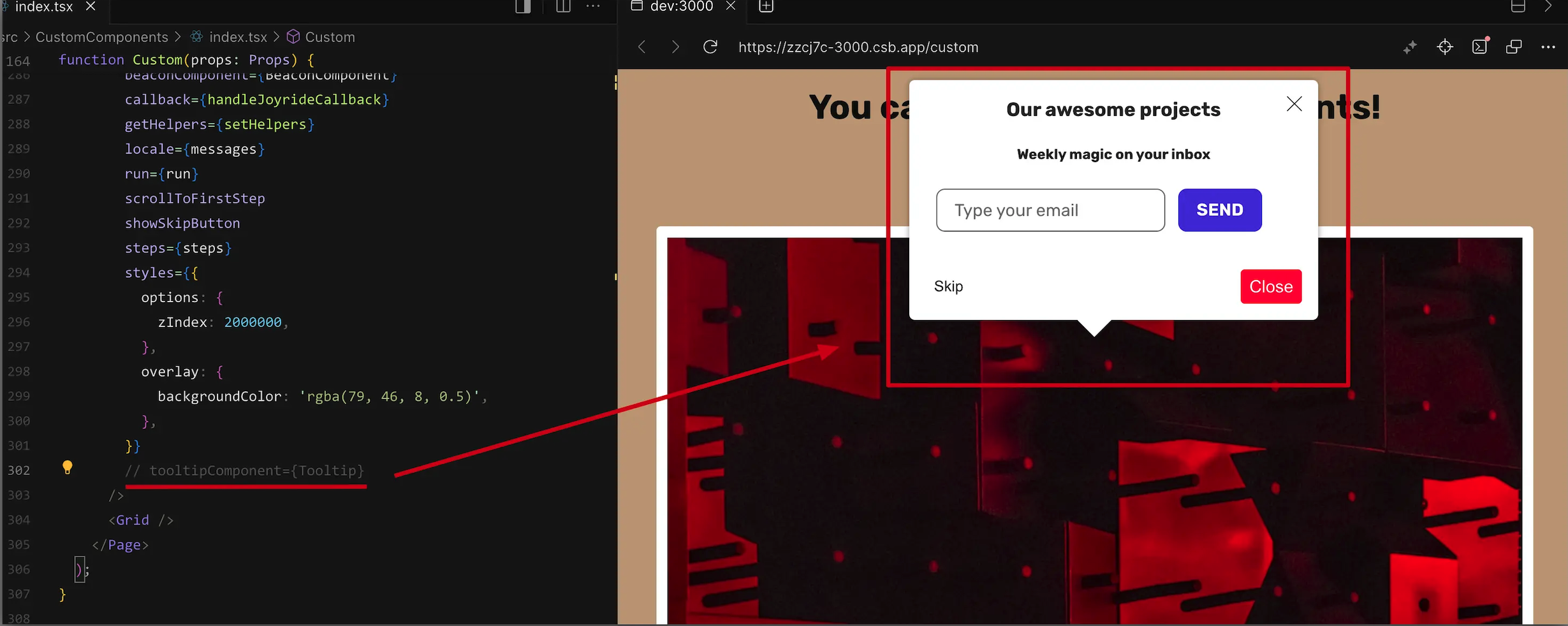
Customizing the Tooltip Component
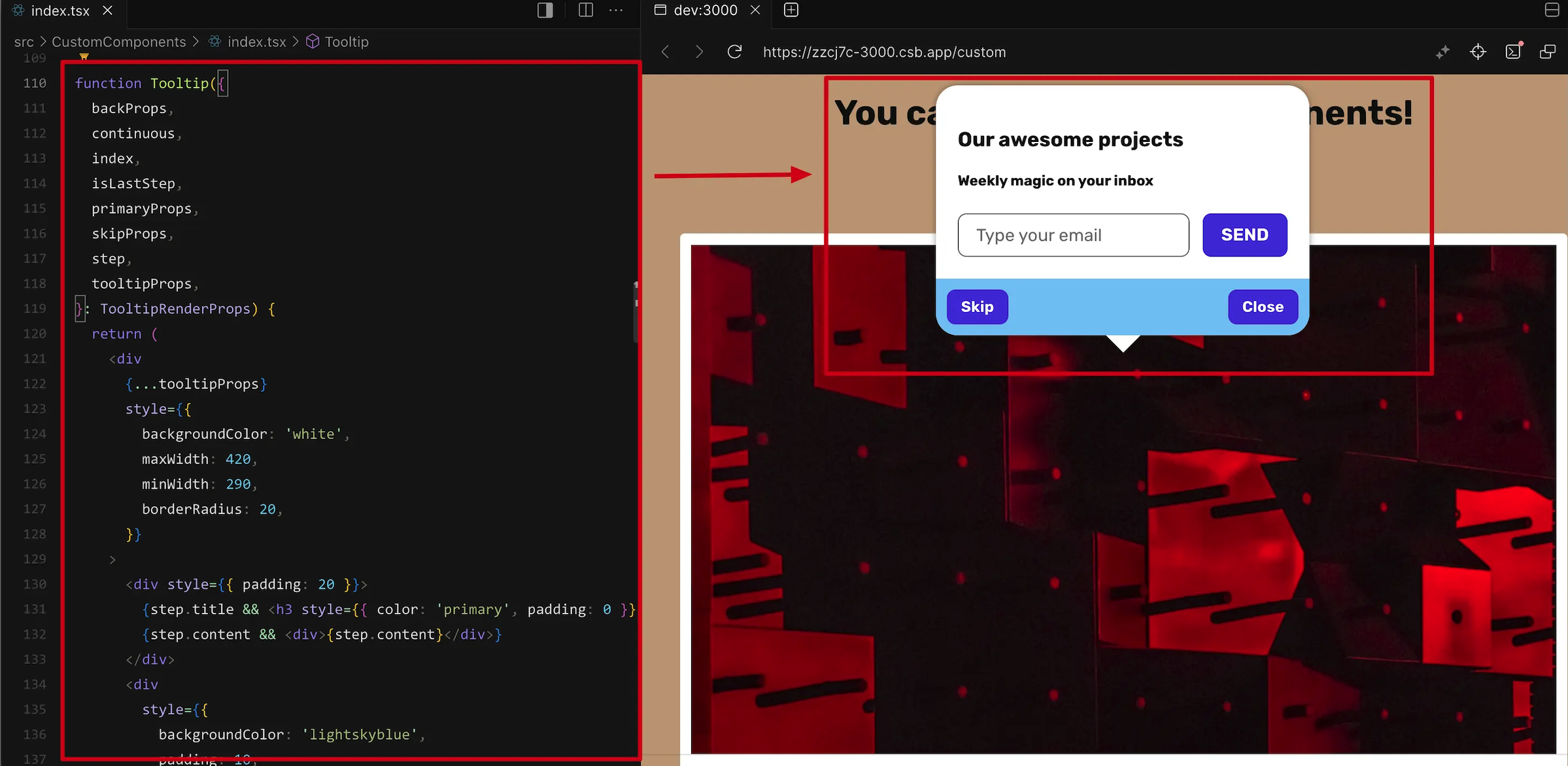
Function implementation code introduction:
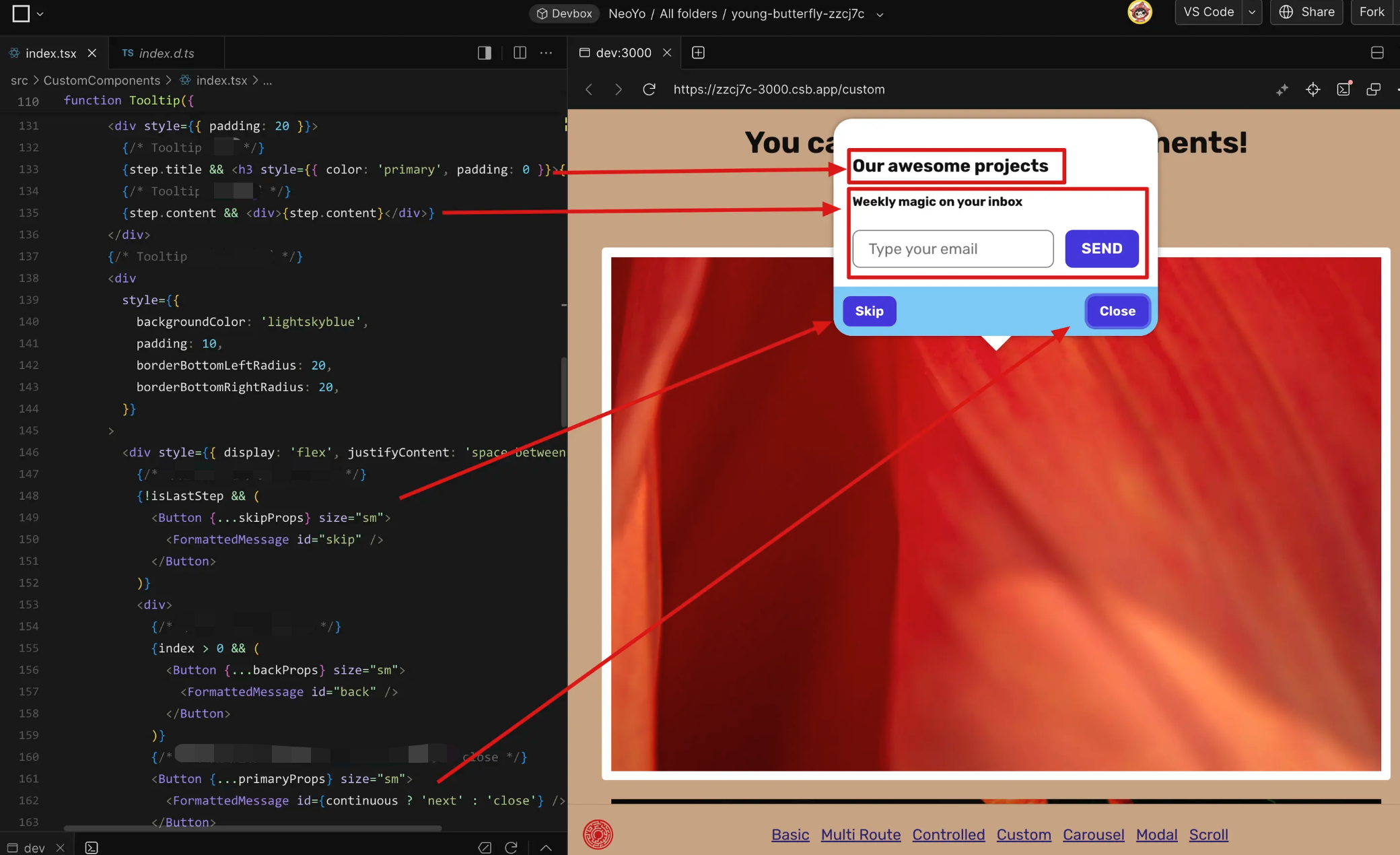
import Joyride, { TooltipRenderProps } from 'react-joyride';
function Tooltip({
backProps,
continuous,
index,
isLastStep,
primaryProps,
skipProps,
step,
tooltipProps,
}: TooltipRenderProps) {
return (
<div
{...tooltipProps}
style={{
backgroundColor: 'white',
maxWidth: 420,
minWidth: 290,
borderRadius: 20,
}}
>
<div style={{ padding: 20 }}>
{step.title && <h3 style={{ color: 'primary', padding: 0 }}>{step.title}</h3>}
{step.content && <div>{step.content}</div>}
</div>
<div
style={{
backgroundColor: 'lightskyblue',
padding: 10,
borderBottomLeftRadius: 20,
borderBottomRightRadius: 20,
}}
>
<div style={{ display: 'flex', justifyContent: 'space-between' }}>
{!isLastStep && (
<Button {...skipProps} size="sm">
<FormattedMessage id="skip" />
</Button>
)}
<div>
{index > 0 && (
<Button {...backProps} size="sm">
<FormattedMessage id="back" />
</Button>
)}
<Button {...primaryProps} size="sm">
<FormattedMessage id={continuous ? 'next' : 'close'} />
</Button>
</div>
</div>
</div>
</div>
);
}
<Joyride
beaconComponent={BeaconComponent}
callback={handleJoyrideCallback}
showSkipButton
steps={steps}
tooltipComponent={Tooltip}
/>;
References
https://docs.react-joyride.com/
https://github.com/gilbarbara/react-joyride
https://github.com/shepherd-pro/shepherd
https://github.com/kamranahmedse/driver.js
https://github.com/usablica/intro.js
https://github.com/elrumordelaluz/reactour \